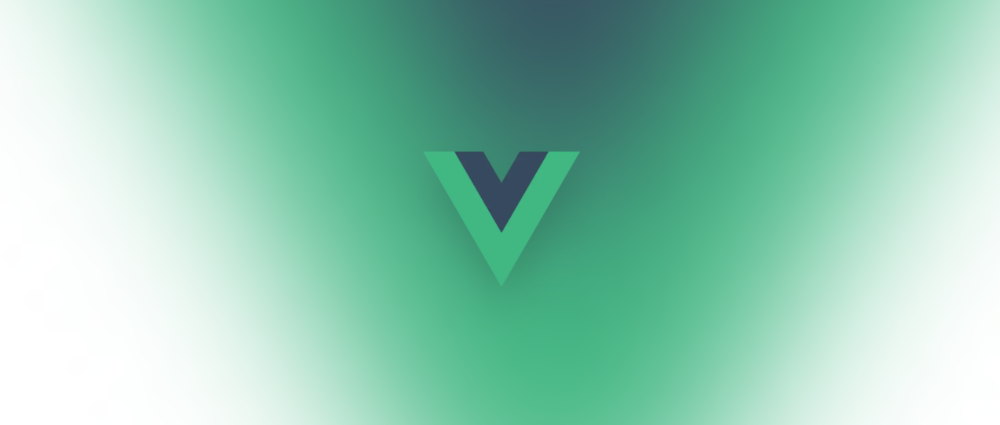
搭建vue开发脚手架
必备知识点以及涉及知识点
- Vue使用基础
- EsLint
- Sass
- Axios
- Css3 transform:scale()
使用官方vue-cli搭建基本框架
1 | $ npm install -g vue-cli |
安装sass预处理
1 | npm install sass-loader node-sass --save-dev |
axios
1 | npm install axios --save |
axios 是一个基于Promise 用于浏览器和 nodejs 的 HTTP 客户端,特点:
- 支持Promise API
- 拦截请求和返回
- 客户端支持抵御XSRF(跨站请求伪造)
中文文档
中文文档2
常见问题
axios默认是请求的时候不会带上cookie的,需要设置withCredentials参数来解决。
1
withCredentials: true
让post使用formdata(大多数Ajax的默认数据格式)格式数据
默认情况下,axios的Post请求参数为JSON格式。
为了发送application/x-wwww-form-urlencoded格式数据,需要使用Qs1
2
3
4
5
6
7// 如果使用npm安装的axios则不用额外安装qs
import Qs from 'qs';
var qs = require('qs');
axios.post('/foo', qs.stringify({'bar':123}));
// 设置Content-Type
axios.defaults.headers.post['Content-Type'] = 'application/x-www-form-urlencoded';带cookie请求
axios默认是发送请求的时候不会带上cookie的,需要通过设置withCredentials: true来解决.
这个时候需要注意需要后端配合设置:
header信息 Access-Control-Allow-Credentials:true
Access-Control-Allow-Origin不可以为**”*”,因为“*”;
会和 Access-Control-Allow-Credentials:true 冲突,需配置指定的地址,如果后端设置 Access-Control-Allow-Origin:“*”**, 会有如下报错信息1
Failed to load http://localhost:8090/category/lists: The value of the 'Access-Control-Allow-Origin' header in the response must not be the wildcard '*' when the request's credentials mode is 'include'. Origin 'http://localhost:8081' is therefore not allowed access. The credentials mode of requests initiated by the XMLHttpRequest is controlled by the withCredentials attribute.
可以参考下之前项目中使用的axios配置
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15import axios from 'axios';
import Qs from 'qs';
const ApiURL = 'http://xxxxx.xx'
Vue.prototype.$ajax = axios.create({
baseURL: ApiURL,
timeout: 5000,
withCredentials: true,
// 这时候我们通过Qs.stringify转换为表单查询参数
transformRequest: [function (data) {
data = Qs.stringify(data);
return data;
}],
// 设置Content-Type
headers: { 'Content-Type': 'application/x-www-form-urlencoded' }
});
项目的其他方面
发布部署问题
1.服务器根目录
把执行npm run build
生成的dist文件夹(不要上传文件夹)里的内容上传到http服务器上就可以了
2.服务器子目录
修改config/index.js
1 | build: { |
添加缩放
为什么要缩放
在移动端页面开发中,要适应多种设备的屏幕尺寸,通过使用CSS3缩放,可以让你直接按照设计图的尺寸去还原.
当然,你也可以使用其他的解决方案,像rem,em等等
原理
通过100vw和100vh的Dom来获取可视区域的宽高
然后把计算与设计稿的缩放比例
把css缩放代码添加到content主内容区
添加meta属性
1 | // 页面的关键字和描述,作者,是写给搜索引擎看的,关键字可以有多个用 ‘,’号隔开 |
EsLint规则
脚手架选用JavaScript Standard Style
normalize.css
选用部分normalize.css重置样式
参考
- 感谢你赐予我前进的力量